مساله:
نقاشی زیر 6 مربع را نشان می دهد که اضلاع آنها دارای طول 1 ، 1 ، 2 ، 3 ، 5 ، 8 است. به راحتی می توان دریافت که مجموع محیط این مربع ها عبارت است از: 4 * (1 + 1 + 2 + 3 + 5 + 8) = 4 * 20 = 80
آیا می توانید مجموع محیط همه مربع ها را در یک مستطیل هنگامی که n + 1 مربع به همان شکل ترسیم شده وجود دارد ، بیان کنید:
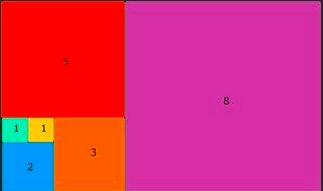
تذکر:
دنباله فیبوناچی را نگاه کنید
تابع محیط دارای پارامتر n است که n + 1 تعداد مربع را نشان می دهد(آنها از 0 تا n شماره گذاری می کنیم) و محیط کلی همه مربع ها را برمی گرداند.
perimeter(5) should return 80 perimeter(7) should return 216
مرجع:
The drawing shows 6 squares the sides of which have a length of 1, 1, 2, 3, 5, 8. It’s easy to see that the sum of the perimeters of these squares is : 4 * (1 + 1 + 2 + 3 + 5 + 8) = 4 * 20 = 80
Could you give the sum of the perimeters of all the squares in a rectangle when there are n + 1 squares disposed in the same manner as in the drawing:
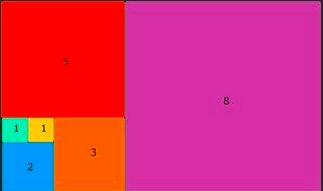
Hint:
See Fibonacci sequence
Ref:
The function perimeter has for parameter n where n + 1 is the number of squares (they are numbered from 0 to n) and returns the total perimeter of all the squares.
perimeter(5) should return 80 perimeter(7) should return 216
import java.math.BigInteger; public class SumFct { public static BigInteger perimeter(BigInteger n) { BigInteger a = BigInteger.ZERO; BigInteger b = BigInteger.ONE; BigInteger c = BigInteger.ONE; BigInteger sum = BigInteger.ZERO; for(int i = 0; i <= n.intValue(); i++) { a = b; b = c; c = a.add(b); sum = sum.add(a); } return sum.multiply(BigInteger.valueOf(4)); } }
import java.math.BigInteger; import java.util.stream.Stream; public class SumFct { public static BigInteger perimeter(BigInteger n) { return Stream.generate(new FibonacciGenerator()::next).limit(n.longValueExact() + 1).reduce(BigInteger::add).get().multiply(BigInteger.valueOf(4)); } private static class FibonacciGenerator { private BigInteger nMinusTwo = BigInteger.ONE; private BigInteger nMinusOne = BigInteger.ZERO; public BigInteger next() { final BigInteger n = nMinusTwo.add(nMinusOne); nMinusTwo = nMinusOne; nMinusOne = n; return n; } } }
import java.math.BigInteger; import java.util.ArrayList; import java.util.List; public class SumFct { public static BigInteger perimeter(BigInteger n) { List<BigInteger> list = new ArrayList<BigInteger>(); BigInteger num1 = BigInteger.valueOf(1), num2 = BigInteger.valueOf(1), sumValue = BigInteger.valueOf(2); list.add(num1); list.add(num2); for (int i = 1; i < n.intValue(); i++) { num1 = num1.add(num2); num2 = num1.subtract(num2); sumValue = sumValue.add(num1); } return sumValue.multiply(BigInteger.valueOf(4)); } }
function perimeter($n) { $fibonacciNumbers = [1,1]; for($i = 2; $i <= $n; $i++){ $fibonacciNumbers[$i] = $fibonacciNumbers[$i-2] + $fibonacciNumbers[$i-1]; } return 4 * array_sum($fibonacciNumbers); }
function perimeter($n) { $sum = 0; $a = 1; $b = 0; $t = 0; while ($n-- >= 0) { $sum += $a; $t = $a; $a += $b; $b = $t; } return 4 * $sum; }
function perimeter($n) { $a = 1; $b = 0; while (3 + $n--) $a = ($b = $a + $b) - $a; return --$b << 2; }